We know that storage classes in embedded c are used to determine the visibility, lifetime , memory location and initial value of a variable.
There are four type of storage classes in embedded c
- Automatic
- External
- Static
- Register
Storage classes | Storage place | Scope | Default Value | Lifetime |
Auto | RAM | Local | Garbage value | Within function |
Extern | RAM | Global | zero | Till the end of program |
Static | RAM | Local | zero | Till the end of program |
Register | Register | Local | Garbage value | Within the function |
Table of Contents
Automatic Storage classes in embedded c
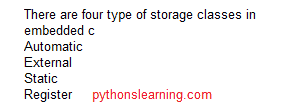
- Automatic variable are allocated memory at runtime
- The lifetime of automatic variable is limited
- Initial value of Automatic variable is garbage
- Every local variable is automatic in c by default.
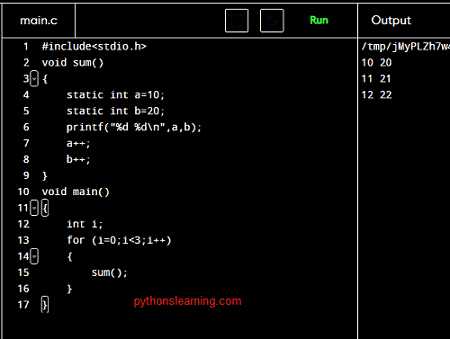
#include<stdio.h>
int main()
{
int a;
char b;
float c;
printf("%d %c %f",a,b,c);
return 0;
}
Output : Garbage value
Static storage classes in embedded c
The variable use as static can hold their value for multiple function call.
same type of static variable can be declared for many time but can be assigned at only one time.
Default initial value of static variable integral variable is 0 otherwise null
The keyword used to define static variable is static
#include<stdio.h>
void sum()
{
static int a=10;
static int b=20;
printf("%d %d\n",a,b);
a++;
b++;
}
void main()
{
int i;
for (i=0;i<3;i++)
{
sum();
}
}
/tmp/jMyPLZh7w4.o
10 20
11 21
12 22
Extern storage classes
The external storage class is used to tell the compiler that the variable defined as extern is declared with an external linkage elsewhere in the program.
The default value of extern variable is zero or null. we can only initialize the extern variable globally
#include<stdio.h>
void sum()
{
extern int a;
//extern int b;
printf("%d\n",a);
a++;
}int a=10;
void main()
{
int i;
for (i=0;i<3;i++)
{
sum();
}
}
/tmp/jMyPLZh7w4.o
10
11
12
Register storage classes
The variable defined as the register is allocated the memory into the CPU register. we cannot use & operator for the register variable
the access time of register is faster as compare to other storage classes.
Register cannot store the address of other variable
#include<stdio.h>
void main()
{
register int a=10;
//extern int b;
printf("%d\n",a);
}
Output : 10
Summary
In this article we saw different storage classes in embedded c with example.