The media player is in dark theme with all the functionalities of a modern media player we can adjust the volume from the player switch back and front to previous and next songs. We can shuffle our playlist and also can move the song to any position as we want using the song slider.
Table of Contents
Which Packages Used for create own Mp3 music player using python
from tkinter import *
import tkinter.ttk as ttk
import pygame
import os
from PIL import ImageTk,Image
import random
from tkinter import filedialog
import time
from mutagen.mp3 import MP3
The Above packages are used to make the make the Media Player
i. tkinter for using Gui in python
ii. tkinter.ttk for using Slider for volume and song track
iii. pygame used for sound libraries
iv. os module for importing songs from file
v. PIL module used for images
vi. Random module used for shuffling playlist
vii. Filedialog used for adding more songs in the player directly
viii. Time module used for tracking song sound
ix. Mutagen used to get the song length
Tk() function is used to initialize the root window. The root window is a main application window in our programs.
Initial after calling all the packages a class My_music_player() is created and in the class a class constructor is created to initialise:
The use of the constructor is given below
i. Initializing Root window
ii. Title of the player
iii. Icon (bitmap)
iv. Dimension(geometry) of the player
v. Initialised pygame and pygame mixer
vi. Variables declared for track and status
vii. Root.root.resizable(0, 0) function is called to prevent resizing of window
viii. Global variables are declared to use in the player
ix. Initializing variables to use it further as accordingly
Media player title and Icon
Play Frame is created to group the control keys of the player.
All the control keys in the player comes under this frame. Control keys include Stop Button, Previous Button, Play and Pause Button,Shuffle Button and Button for hiding the Playlist.
Control Keys for MP3 player in python
All the Buttons are Assigned their respective functions using command attribute. The functionalities are stop button for stopping the music, Previous and Next Button for Previous and next songs, Play and Pause Button for playing and pausing the musing, shuffle button is also included to shuffle the play list.
The button keys are made using icon and Font Awesome 5 font.
The play pause button chances according to the playing and pausing of music
Change in Play Pause Button in python
Buttons and Lables of Tkinter module is used to create the control key in the play frame.
Playerarea Frame is created to show the player area where Image is show for better looks.
Song slider and volume controls are given in this frame.
Song Description and status of the song is also included in this frame.
The duration of the song and time elapsed is also shown in this frame.
Volume control functions in MP3 player in python
The symbol of the volume icon changes on increasing and decreasing of the volume and mute and unmute the volume.
Volume can be adjusted using the slider scrolling up and down
Ttk.scale() is used to create the volume slider.
Functions and attributes of Pygame module are use to adjust and set the volume using the slider
We can move the song to any position as we want using the song slider.
The Description area shows the Name of the song currently Playing and also the status of the song i.e. Playing, Paused or Stopped.
Add Button in MP3 player in python
Add Button is also included to add song to the player directly form the computer we can select multiple files also at once. The songs are added at the end of the playlist.
The Player Frame with above mentioned fuctionalities
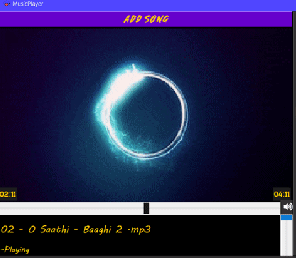
The third Frame used in this media player is song_list Frame.
This frame is used for the playlist of the media player.
Listbox of tkinter is used for the playlist.
Scrolling option on the right side of the playlist is enabled for scrolling along y axis to access songs in the playlist.
Double click functionality is enables on the listbox to play the selected song.
Song can also be played using tha play button after selecting the song by clicking once.
Bind() is use to bind the mouse click for playing songs.
Functions of OS modules are used to fetch the song from the song folder.
Song can also be added using the add button.
Many function are created to do task according to the button press from the control area. The functions include function to add song, function to manage double click and playing the song, function to adjust volume, Functions for play and pause music and start and stop music, function to hide playlist, function to shuffle the playlist, functions to mute and unmute.
Functionalities for previous and next songs.and slider functions.
The whole media player looks like this
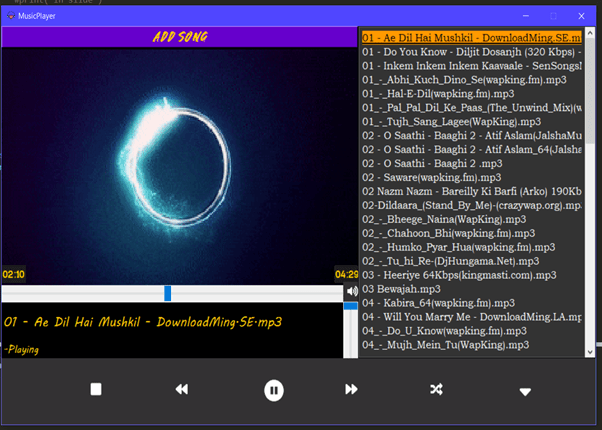
Source Coding For Create Mp3 music player in python :
We need to First import all necessary Library
from tkinter import *
from tkinter import filedialog
from pygame import mixer
Create class & Buttons for our application
class MusicPlayer:
def __init__(self, window ):
window.geometry('320x100'); window.title('Iris Player'); window.resizable(0,0)
Load = Button(window, text = 'Load', width = 10, font = ('Times', 10), command = self.load)
Play = Button(window, text = 'Play', width = 10,font = ('Times', 10), command = self.play)
Pause = Button(window,text = 'Pause', width = 10, font = ('Times', 10), command = self.pause)
Stop = Button(window ,text = 'Stop', width = 10, font = ('Times', 10), command = self.stop)
Load.place(x=0,y=20);Play.place(x=110,y=20);Pause.place(x=220,y=20);Stop.place(x=110,y=60)
self.music_file = False
self.playing_state = False
Adding Load Method to our MusicPlayer class
class MusicPlayer:
def __init__(self, window ):
window.geometry('320x100'); window.title('Iris Player'); window.resizable(0,0)
Load = Button(window, text = 'Load', width = 10, font = ('Times', 10), command = self.load)
Play = Button(window, text = 'Play', width = 10,font = ('Times', 10), command = self.play)
Pause = Button(window,text = 'Pause', width = 10, font = ('Times', 10), command = self.pause)
Stop = Button(window ,text = 'Stop', width = 10, font = ('Times', 10), command = self.stop)
Load.place(x=0,y=20);Play.place(x=110,y=20);Pause.place(x=220,y=20);Stop.place(x=110,y=60)
self.music_file = False
self.playing_state = False
def load(self):
self.music_file = filedialog.askopenfilename()
Adding Play Method to our class
class MusicPlayer:
def __init__(self, window ):
window.geometry('320x100'); window.title('Iris Player'); window.resizable(0,0)
Load = Button(window, text = 'Load', width = 10, font = ('Times', 10), command = self.load)
Play = Button(window, text = 'Play', width = 10,font = ('Times', 10), command = self.play)
Pause = Button(window,text = 'Pause', width = 10, font = ('Times', 10), command = self.pause)
Stop = Button(window ,text = 'Stop', width = 10, font = ('Times', 10), command = self.stop)
Load.place(x=0,y=20);Play.place(x=110,y=20);Pause.place(x=220,y=20);Stop.place(x=110,y=60)
self.music_file = False
self.playing_state = False
def load(self):
self.music_file = filedialog.askopenfilename()
def play(self):
if self.music_file:
mixer.init()
mixer.music.load(self.music_file)
mixer.music.play()
After adding Play Method to our class we need a Method to pause and unpause & also to stop the Music
Finally Let’s add pause and stop method to our class
Python
class MusicPlayer:
def __init__(self, window ):
window.geometry('320x100'); window.title('Iris Player'); window.resizable(0,0)
Load = Button(window, text = 'Load', width = 10, font = ('Times', 10), command = self.load)
Play = Button(window, text = 'Play', width = 10,font = ('Times', 10), command = self.play)
Pause = Button(window,text = 'Pause', width = 10, font = ('Times', 10), command = self.pause)
Stop = Button(window ,text = 'Stop', width = 10, font = ('Times', 10), command = self.stop)
Load.place(x=0,y=20);Play.place(x=110,y=20);Pause.place(x=220,y=20);Stop.place(x=110,y=60)
self.music_file = False
self.playing_state = False
def load(self):
self.music_file = filedialog.askopenfilename()
def play(self):
if self.music_file:
mixer.init()
mixer.music.load(self.music_file)
mixer.music.play()
def pause(self):
if not self.playing_state:
mixer.music.pause()
self.playing_state=True
else:
mixer.music.unpause()
self.playing_state = False
def stop(self):
mixer.music.stop()
look at our Final will app (app.py)
from tkinter import *
from tkinter import filedialog
from pygame import mixer
class MusicPlayer:
def __init__(self, window ):
window.geometry('320x100'); window.title('Iris Player'); window.resizable(0,0)
Load = Button(window, text = 'Load', width = 10, font = ('Times', 10), command = self.load)
Play = Button(window, text = 'Play', width = 10,font = ('Times', 10), command = self.play)
Pause = Button(window,text = 'Pause', width = 10, font = ('Times', 10), command = self.pause)
Stop = Button(window ,text = 'Stop', width = 10, font = ('Times', 10), command = self.stop)
Load.place(x=0,y=20);Play.place(x=110,y=20);Pause.place(x=220,y=20);Stop.place(x=110,y=60)
self.music_file = False
self.playing_state = False
def load(self):
self.music_file = filedialog.askopenfilename()
def play(self):
if self.music_file:
mixer.init()
mixer.music.load(self.music_file)
mixer.music.play()
def pause(self):
if not self.playing_state:
mixer.music.pause()
self.playing_state=True
else:
mixer.music.unpause()
self.playing_state = False
def stop(self):
mixer.music.stop()
root = Tk()
app= MusicPlayer(root)
root.mainloop()
Summary :
In this article we saw how to make your own MP3 music player in python using Tkinter so about this article you have any query then free to ask me